Tree and Linked List Data Structure
July 10, 2015
SQL (Structured Query Language)
SQL is used to communicate with a relational database. The following are the most frequently used command.
Let's look at 'GROUP BY'
Table Name: Store_Information
Store_Name | Sales | Txn_Date |
Los Angeles | 1500 | Jan-05-1999 |
San Diego | 250 | Jan-07-1999 |
Los Angeles | 300 | Jan-08-1999 |
Boston | 700 | Jan-08-1999 |
Los Angeles | 1800 |
San Diego | 250 |
Boston | 700 |
Inner Join(Common Element), Outer Join(Everything) and Left and Right
Inner Join
Let's say we have a two column a and b. a has 1, 2, 3, 4 and b has 3, 4, 5, 6. Inner join is to find the same element from the each column. So it will return 3, 4 the common element from the both column.
Left Outer Join
First, don't be intimidated by 'left' side of it. Left always means that it is the anchor of the other columns. In our above example, 'a' is going to be an 'left' side of it. Left Outer Join returns everything from 'left' side (so it will be 'a' column) and any common element from other side of it. So it will return 1, 2, 3, 4 from a and 3, 4 from b.
Full Outer Join
This is very generous joining. This will return everything from a and b. It is also notable that this will not only return every individual element but it will match the number of elements and return null value for the columns that empty. For instance, if a has null, null, 1, 2, 3, 4 and b has 3, 4, 5, 6, null, null, Full Outer Join will return null, null, 1, 2, 3, 4, 3, 4, 5, 6, null, null.
Left and Right Join
Left Join will return all elements from left and nothing from right. Right join will return every elements from right column. This join will naturally returns common elements from other side.
Full details about join sql command can be found in here
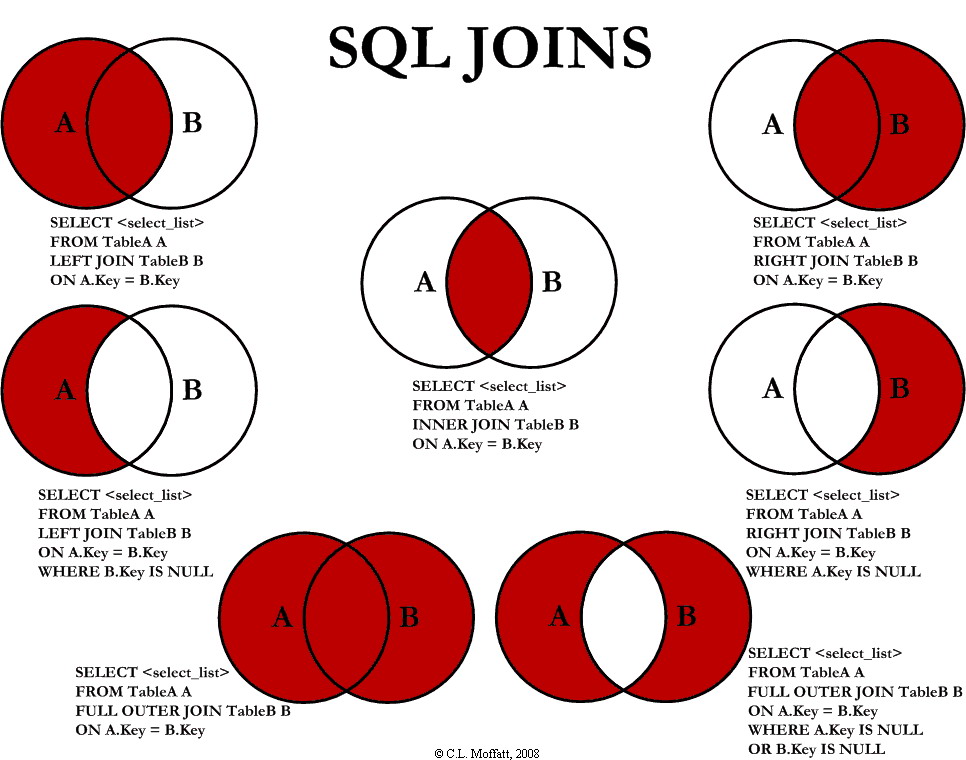